

Support the Author: Buy the book on Amazon or
the book/ebook bundle directly from No Starch Press.

Read the author's other free Python books:
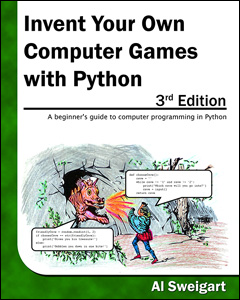
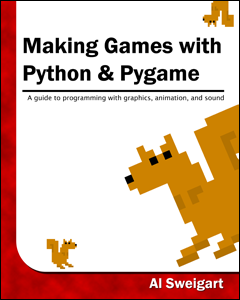
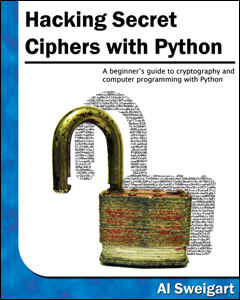
This appendix contains the answers to the practice problems at the end of each chapter. I highly recommend that you take the time to work through these problems. Programming is more than memorizing syntax and a list of function names. As when learning a foreign language, the more practice you put into it, the more you will get out of it. There are many websites with practice programming problems as well. You can find a list of these at http://nostarch.com/automatestuff/.
-
The operators are
+
,-
,*
, and/
. The values are'hello'
,-88.8
, and5
. -
The string is
'spam'
; the variable isspam
. Strings always start and end with quotes. -
The three data types introduced in this chapter are integers, floating-point numbers, and strings.
-
An expression is a combination of values and operators. All expressions evaluate (that is, reduce) to a single value.
-
An expression evaluates to a single value. A statement does not.
-
The
bacon
variable is set to20
. Thebacon + 1
expression does not reassign the value inbacon
(that would need an assignment statement:bacon = bacon + 1
). -
Both expressions evaluate to the string
'spamspamspam'
. -
Variable names cannot begin with a number.
-
The
int()
,float()
, andstr(
) functions will evaluate to the integer, floating-point number, and string versions of the value passed to them. -
The expression causes an error because
99
is an integer, and only strings can be concatenated to other strings with the+
operator. The correct way isI have eaten ' + str(99) + ' burritos.'
.
-
True
andFalse
, using capital T and F, with the rest of the word in lowercase -
and
,or
, andnot
-
True and True
isTrue
.True and False
isFalse
.False and True
isFalse
.False and False
isFalse
.True or True
isTrue
.True or False
isTrue
.False or True
isTrue
.False or False
isFalse
.not True
isFalse
.not False
isTrue
. -
False False True False False True
-
==
,!=
,<
,>
,<=
, and>=
. -
==
is the equal to operator that compares two values and evaluates to a Boolean, while=
is the assignment operator that stores a value in a variable. -
A condition is an expression used in a flow control statement that evaluates to a Boolean value.
-
The three blocks are everything inside the
if
statement and the linesprint('bacon')
andprint('ham')
.print('eggs') if spam > 5: print('bacon') else: print('ham') print('spam')
-
The code:
if spam == 1: print('Hello') elif spam == 2: print('Howdy') else: print('Greetings!')
-
Press CTRL-C to stop a program stuck in an infinite loop.
-
The
break
statement will move the execution outside and just after a loop. Thecontinue
statement will move the execution to the start of the loop. -
They all do the same thing. The
range(10)
call ranges from0
up to (but not including)10
,range(0, 10)
explicitly tells the loop to start at0
, andrange(0, 10, 1)
explicitly tells the loop to increase the variable by1
on each iteration. -
The code:
for i in range(1, 11): print(i)
and:
i = 1 while i <= 10: print(i) i = i + 1
-
This function can be called with
spam.bacon()
.
-
Functions reduce the need for duplicate code. This makes programs shorter, easier to read, and easier to update.
-
The code in a function executes when the function is called, not when the function is defined.
-
The
def
statement defines (that is, creates) a function. -
A function consists of the
def
statement and the code in itsdef
clause.A function call is what moves the program execution into the function, and the function call evaluates to the function’s return value.
-
There is one global scope, and a local scope is created whenever a function is called.
-
When a function returns, the local scope is destroyed, and all the variables in it are forgotten.
-
A return value is the value that a function call evaluates to. Like any value, a return value can be used as part of an expression.
-
If there is no return statement for a function, its return value is
None
. -
A
global
statement will force a variable in a function to refer to the global variable. -
The data type of
None
isNoneType
. -
That
import
statement imports a module namedareallyourpetsnamederic
. (This isn’t a real Python module, by the way.) -
This function can be called with
spam.bacon()
. -
Place the line of code that might cause an error in a
try
clause. -
The code that could potentially cause an error goes in the
try
clause.The code that executes if an error happens goes in the
except
clause.
-
The empty list value, which is a list value that contains no items. This is similar to how
''
is the empty string value. -
spam[2] = 'hello'
(Notice that the third value in a list is at index2
because the first index is0
.) -
'd'
(Note that'3' * 2
is the string'33'
, which is passed toint()
before being divided by11
. This eventually evaluates to3
. Expressions can be used wherever values are used.) -
'd'
(Negative indexes count from the end.) -
['a', 'b']
-
1
-
[3.14, 'cat', 11, 'cat', True, 99]
-
[3.14, 11, 'cat', True]
-
The operator for list concatenation is
+
, while the operator for replication is*
. (This is the same as for strings.) -
While
append()
will add values only to the end of a list,insert()
can add them anywhere in the list. -
The
del
statement and theremove()
list method are two ways to remove values from a list. -
Both lists and strings can be passed to
len()
, have indexes and slices, be used infor
loops, be concatenated or replicated, and be used with thein
andnot in
operators. -
Lists are mutable; they can have values added, removed, or changed. Tuples are immutable; they cannot be changed at all. Also, tuples are written using parentheses,
(
and)
, while lists use the square brackets,[
and]
. -
(42,)
(The trailing comma is mandatory.) -
The
tuple()
andlist()
functions, respectively -
They contain references to list values.
-
The
copy.copy()
function will do a shallow copy of a list, while thecopy.deepcopy()
function will do a deep copy of a list. That is, onlycopy.deepcopy()
will duplicate any lists inside the list.
-
Two curly brackets:
{}
-
{'foo': 42}
-
The items stored in a dictionary are unordered, while the items in a list are ordered.
-
You get a
KeyError
error. -
There is no difference. The
in
operator checks whether a value exists as a key in the dictionary. -
'cat' in spam
checks whether there is a'cat'
key in the dictionary, while'cat' in spam.values()
checks whether there is a value'cat'
for one of the keys inspam
. -
spam.setdefault('color', 'black')
-
pprint.pprint()
-
Escape characters represent characters in string values that would otherwise be difficult or impossible to type into code.
-
\n
is a newline;\t
is a tab. -
The
\\
escape character will represent a backslash character. -
The single quote in
Howl's
is fine because you’ve used double quotes to mark the beginning and end of the string. -
Multiline strings allow you to use newlines in strings without the
\n
escape character. -
The expressions evaluate to the following:
-
'e'
-
'Hello'
-
'Hello'
-
'lo world!
-
-
The expressions evaluate to the following:
-
'HELLO'
-
True
-
'hello'
-
-
The expressions evaluate to the following:
-
['Remember,', 'remember,', 'the', 'fifth', 'of', 'November.']
-
'There-can-be-only-one.'
-
-
The
rjust()
,ljust()
, andcenter()
string methods, respectively -
The
lstrip()
andrstrip()
methods remove whitespace from the left and right ends of a string, respectively.
-
The
re.compile()
function returnsRegex
objects. -
Raw strings are used so that backslashes do not have to be escaped.
-
The
search()
method returnsMatch
objects. -
The
group()
method returns strings of the matched text. -
Group
0
is the entire match, group1
covers the first set of parentheses, and group2
covers the second set of parentheses. -
Periods and parentheses can be escaped with a backslash:
\.
,\(
, and\)
. -
If the regex has no groups, a list of strings is returned. If the regex has groups, a list of tuples of strings is returned.
-
The
|
character signifies matching “either, or” between two groups. -
The
?
character can either mean “match zero or one of the preceding group” or be used to signify nongreedy matching. -
The
+
matches one or more. The*
matches zero or more. -
The
{3}
matches exactly three instances of the preceding group. The{3,5}
matches between three and five instances. -
The
\d
,\w
, and\s
shorthand character classes match a single digit, word, or space character, respectively. -
The
\D
,\W
, and\S
shorthand character classes match a single character that is not a digit, word, or space character, respectively. -
Passing
re.I
orre.IGNORECASE
as the second argument tore.compile()
will make the matching case insensitive. -
The
.
character normally matches any character except the newline character. Ifre.DOTALL
is passed as the second argument tore.compile()
, then the dot will also match newline characters. -
The
.*
performs a greedy match, and the.*?
performs a nongreedy match. -
Either
[0-9a-z]
or[a-z0-9]
-
'X drummers, X pipers, five rings, X hens'
-
The
re.VERBOSE
argument allows you to add whitespace and comments to the string passed tore.compile()
. -
re.compile(r'^\d{1,3}(,\d{3})*$')
will create this regex, but other regex strings can produce a similar regular expression. -
re.compile(r'[A-Z][a-z]*\sNakamoto')
-
re.compile(r'(Alice|Bob|Carol)\s(eats|pets|throws)\s(apples|cats|baseballs)\.', re.IGNORECASE)
-
Relative paths are relative to the current working directory.
-
Absolute paths start with the root folder, such as / or C:\.
-
The
os.getcwd()
function returns the current working directory. Theos.chdir()
function changes the current working directory. -
The
.
folder is the current folder, and..
is the parent folder. -
C:\bacon\eggs is the dir name, while spam.txt is the base name.
-
The string
'r'
for read mode,'w'
for write mode, and'a'
for append mode -
An existing file opened in write mode is erased and completely overwritten.
-
The
read()
method returns the file’s entire contents as a single string value. Thereadlines()
method returns a list of strings, where each string is a line from the file’s contents. -
A shelf value resembles a dictionary value; it has keys and values, along with
keys()
andvalues()
methods that work similarly to the dictionary methods of the same names.
-
The
shutil.copy()
function will copy a single file, whileshutil.copytree()
will copy an entire folder, along with all its contents. -
The
shutil.move()
function is used for renaming files, as well as moving them. -
The
send2trash
functions will move a file or folder to the recycle bin, whileshutil
functions will permanently delete files and folders. -
The
zipfile.ZipFile()
function is equivalent to theopen()
function; the first argument is the filename, and the second argument is the mode to open the ZIP file in (read, write, or append).
-
assert spam >= 10, 'The spam variable is less than 10.'
-
assert eggs.lower() != bacon.lower(), 'The eggs and bacon variables are the same!'
orassert eggs.upper() != bacon.upper(), 'The eggs and bacon variables are the same!'
-
assert False, 'This assertion always triggers.'
-
To be able to call
logging.debug()
, you must have these two lines at the start of your program:import logging logging.basicConfig(level=logging.DEBUG, format=' %(asctime)s - %(levelname)s - %(message)s')
-
To be able to send logging messages to a file named programLog.txt with
logging.debug()
, you must have these two lines at the start of your program:import logging >>> logging.basicConfig(filename='programLog.txt', level=logging.DEBUG, format=' %(asctime)s - %(levelname)s - %(message)s')
-
DEBUG, INFO, WARNING, ERROR, and CRITICAL
-
logging.disable(logging.CRITICAL)
-
You can disable logging messages without removing the logging function calls. You can selectively disable lower-level logging messages. You can create logging messages. Logging messages provides a timestamp.
-
The Step button will move the debugger into a function call. The Over button will quickly execute the function call without stepping into it. The Out button will quickly execute the rest of the code until it steps out of the function it currently is in.
-
After you click Go, the debugger will stop when it has reached the end of the program or a line with a breakpoint.
-
A breakpoint is a setting on a line of code that causes the debugger to pause when the program execution reaches the line.
-
To set a breakpoint in IDLE, right-click the line and select Set Breakpoint from the context menu.
-
The
webbrowser
module has anopen()
method that will launch a web browser to a specific URL, and that’s it. Therequests
module can download files and pages from the Web. TheBeautifulSoup
module parses HTML. Finally, theselenium
module can launch and control a browser. -
The
requests.get()
function returns aResponse
object, which has atext
attribute that contains the downloaded content as a string. -
The
raise_for_status()
method raises an exception if the download had problems and does nothing if the download succeeded. -
The
status_code
attribute of theResponse
object contains the HTTP status code. -
After opening the new file on your computer in
'wb'
“write binary” mode, use afor
loop that iterates over theResponse
object’siter_content()
method to write out chunks to the file. Here’s an example:saveFile = open('filename.html', 'wb') for chunk in res.iter_content(100000): saveFile.write(chunk)
-
F12 brings up the developer tools in Chrome. Pressing CTRL-SHIFT-C (on Windows and Linux) or ⌘-OPTION-C (on OS X) brings up the developer tools in Firefox.
-
Right-click the element in the page, and select Inspect Element from the menu.
-
'#main'
-
'.highlight'
-
'div div'
-
'button[value="favorite"]'
-
spam.getText()
-
linkElem.attrs
-
The
selenium
module is imported withfrom selenium import webdriver
. -
The
find_element_*
methods return the first matching element as aWebElement
object. Thefind_elements_*
methods return a list of all matching elements asWebElement
objects. -
The
click()
andsend_keys()
methods simulate mouse clicks and keyboard keys, respectively. -
Calling the
submit()
method on any element within a form submits the form. -
The
forward()
,back()
, andrefresh() WebDriver
object methods simulate these browser buttons.
-
The
openpyxl.load_workbook()
function returns aWorkbook
object. -
The
get_sheet_names()
method returns aWorksheet
object. -
Call
wb.get_sheet_by_name('Sheet1')
. -
Call
wb.get_active_sheet()
. -
sheet['C5'].value
orsheet.cell(row=5, column=3).value
-
sheet['C5'] = 'Hello'
orsheet.cell(row=5, column=3).value = 'Hello'
-
cell.row
andcell.column
-
They return the highest column and row with values in the sheet, respectively, as integer values.
-
openpyxl.cell.column_index_from_string('M')
-
openpyxl.cell.get_column_letter(14)
-
sheet['A1':'F1']
-
wb.save('example.xlsx’)
-
A formula is set the same way as any value. Set the cell’s
value
attribute to a string of the formula text. Remember that formulas begin with the = sign. -
sheet.row_dimensions[5].height = 100
-
sheet.column_dimensions['C'].hidden = True
-
OpenPyXL 2.0.5 does not load freeze panes, print titles, images, or charts.
-
Freeze panes are rows and columns that will always appear on the screen. They are useful for headers.
-
openpyxl.charts.Reference()
,openpyxl.charts.Series()
,openpyxl.charts. BarChart()
,chartObj.append(seriesObj)
, andadd_chart()
-
A
File
object returned fromopen()
-
Read-binary (
'rb'
) forPdfFileReader()
and write-binary ('wb'
) forPdfFileWriter()
-
Calling
getPage(4)
will return aPage
object for About This Book, since page 0 is the first page. -
The
numPages
variable stores an integer of the number of pages in thePdfFileReader
object. -
Call
decrypt('swordfish')
. -
The
rotateClockwise()
androtateCounterClockwise()
methods. The degrees to rotate is passed as an integer argument. -
docx.Document('demo.docx')
-
A document contains multiple paragraphs. A paragraph begins on a new line and contains multiple runs. Runs are contiguous groups of characters within a paragraph.
-
Use
doc.paragraphs
. -
A
Run
object has these variables (not a Paragraph). -
True
always makes theRun
object bolded andFalse
makes it always not bolded, no matter what the style’s bold setting is.None
will make theRun
object just use the style’s bold setting. -
Call the
docx.Document()
function. -
doc.add_paragraph('Hello there!')
-
The integers
0
,1
,2
,3
, and4
-
In Excel, spreadsheets can have values of data types other than strings; cells can have different fonts, sizes, or color settings; cells can have varying widths and heights; adjacent cells can be merged; and you can embed images and charts.
-
You pass a
File
object, obtained from a call toopen()
. -
File
objects need to be opened in read-binary ('rb'
) forReader
objects and write-binary ('wb'
) forWriter
objects. -
The
writerow()
method -
The
delimiter
argument changes the string used to separate cells in a row. Thelineterminator
argument changes the string used to separate rows. -
json.loads()
-
json.dumps()
-
A reference moment that many date and time programs use. The moment is January 1st, 1970, UTC.
-
time.time()
-
time.sleep(5)
-
It returns the closest integer to the argument passed. For example,
round(2.4)
returns2
. -
A
datetime
object represents a specific moment in time. Atimedelta
object represents a duration of time. -
threadObj = threading.Thread(target=spam)
-
threadObj.start()
-
Make sure that code running in one thread does not read or write the same variables as code running in another thread.
-
subprocess.Popen('c:\\Windows\\System32\\calc.exe')
-
SMTP and IMAP, respectively
-
smtplib.SMTP()
,smtpObj.ehlo()
,smptObj.starttls()
, andsmtpObj.login()
-
imapclient.IMAPClient()
andimapObj.login()
-
A list of strings of IMAP keywords, such as
'BEFORE <date>'
,'FROM <string>'
, or'SEEN'
-
Assign the variable
imaplib._MAXLINE
a large integer value, such as10000000
. -
The
pyzmail
module reads downloaded emails. -
You will need the Twilio account SID number, the authentication token number, and your Twilio phone number.
-
An RGBA value is a tuple of 4 integers, each ranging from 0 to 255. The four integers correspond to the amount of red, green, blue, and alpha (transparency) in the color.
-
A function call to
ImageColor.getcolor('CornflowerBlue', 'RGBA')
will return(100, 149, 237, 255)
, the RGBA value for that color. -
A box tuple is a tuple value of four integers: the left edge x-coordinate, the top edge y-coordinate, the width, and the height, respectively.
-
Image.open('zophie.png')
-
imageObj.size
is a tuple of two integers, the width and the height. -
imageObj.crop((0, 50, 50, 50))
. Notice that you are passing a box tuple tocrop()
, not four separate integer arguments. -
Call the
imageObj.save('new_filename.png')
method of theImage
object. -
The
ImageDraw
module contains code to draw on images. -
ImageDraw
objects have shape-drawing methods such aspoint()
,line()
, orrectangle()
. They are returned by passing theImage
object to theImageDraw.Draw()
function.
-
Move the mouse to the top-left corner of the screen, that is, the (0, 0) coordinates.
-
pyautogui.size()
returns a tuple with two integers for the width and height of the screen. -
pyautogui.position()
returns a tuple with two integers for the x- and y-coordinates of the mouse cursor. -
The
moveTo()
function moves the mouse to absolute coordinates on the screen, while themoveRel()
function moves the mouse relative to the mouse’s current position. -
pyautogui.dragTo()
andpyautogui.dragRel()
-
pyautogui.typewrite('Hello world!')
-
Either pass a list of keyboard key strings to
pyautogui.typewrite()
(such as'left'
) or pass a single keyboard key string topyautogui.press()
. -
pyautogui.screenshot('screenshot.png')
-
pyautogui.PAUSE = 2

